Hallo,
ich hab ein kleines Problem bei c#
Ich möchte die Daten eines Konstruktors in ein 2d-Feld übergeben ohne von Hand die Indizes hochzuzählen.
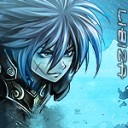
C# Parameter in 2d Array
-
-
-
Bin mir jetzt nicht ganz sicher was du meinst, aber 2D Array befüllen geht i.D.R so:
CodeNur so als kleinen Denkansatz, weiß nicht genau ob das das ist was du suchst
-
Vielen Dank für deine Antwort.
Ich hab jetzt ein Doublearray im Konstruktor übergeben und den Index hochgezählt und jeweils dem nächsten Element zugewiesen. Wenn du willlst kann ich es dir hochladen. -
-
Die Lösung ist immer interessant
-
Bitte
Code- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- namespace OperatorOverloadPraktikum3
- {
- class CMatrix
- {
- double[,] Matrix = new double[3, 3];
- public CMatrix(double[] da)
- {
- int index = 0;
- for (int i = 0; i < Matrix.GetLength(0); i++)
- {
- for (int j = 0; j < Matrix.GetLength(1); j++)
- {
- Matrix[i, j] = da[index];
- index++;
- }
- }
- }
- // Konstruktor der Einheitsmatrix
- public CMatrix()
- {
- for (int i = 0; i < Matrix.GetLength(0); i++)
- {
- for (int j = 0; j < Matrix.GetLength(1); j++)
- {
- if (i == j) // Trägt in der Diagonalen 1.0 ein, sonst 0.0
- Matrix[i, j] = 1.0;
- else
- Matrix[i, j] = 0.0;
- }
- }
- }
- public void GebeWerteAus()
- {
- int Spalten = 0;
- foreach (double item in Matrix)
- {
- Console.Write("{0, -10} ", item); // die -10 lässt 10 Zeichen frei
- Spalten++;
- if (Spalten > Matrix.GetLength(0)-1)
- {
- // Formatiert die Ausgabe der Zeilen
- Console.WriteLine("\n");
- Spalten = 0;
- }
- }
- }
- public static CMatrix operator +(CMatrix m1, CMatrix m2) // Addition zweier Matrizen
- {
- CMatrix m3 = new CMatrix();
- for (int i = 0; i < 3; i++)
- {
- for (int j = 0; j < 3; j++)
- {
- m3.Matrix[i, j] = m1.Matrix[i, j] + m2.Matrix[i, j];
- }
- }
- return m3;
- }
- public static CMatrix operator *(CMatrix m1, CMatrix m2) // Multiplikation zweier Matrizen
- {
- CMatrix m3 = new CMatrix();
- for (int k = 0; k < 3; k++)
- {
- for (int i = 0; i < 3; i++)
- {
- double summe = 0.0;
- for (int j = 0; j < 3; j++)
- {
- summe = summe + (m1.Matrix[i, j] * m2.Matrix[j, k]);
- }
- m3.Matrix[i,k] = summe;
- }
- }
- return m3;
- }
- public static CMatrix operator *(CMatrix m1, int Skalar) //Multiplikation mit Skalar
- {
- CMatrix m3 = new CMatrix();
- for (int i = 0; i < 3; i++)
- {
- for (int j = 0; j < 3; j++)
- {
- m3.Matrix[i, j] = m1.Matrix[i, j] * Skalar;
- }
- }
- return m3;
- }
- public static explicit operator double(CMatrix m1) // Saurus-Regel wird angewandt
- {
- return
- m1.Matrix[0, 0] * m1.Matrix[1, 1] * m1.Matrix[2, 2]
- + m1.Matrix[0, 1] * m1.Matrix[1, 2] * m1.Matrix[2, 0]
- + m1.Matrix[0, 2] * m1.Matrix[1, 0] * m1.Matrix[2, 1]
- - m1.Matrix[0, 2] * m1.Matrix[1, 1] * m1.Matrix[2, 0]
- - m1.Matrix[1, 2] * m1.Matrix[2, 1] * m1.Matrix[0, 0]
- - m1.Matrix[2, 2] * m1.Matrix[0, 1] * m1.Matrix[1, 0];
- }
- }
- class Program
- {
- static void Main(string[] args)
- {
- double[] da1 = new double[] { 2.3, -1.5, 6.3, -4.4, 9.2, 2.3, 6.2, -4.5, 7.6 };
- double[] da2 = new double[] { 7.3, 4.6, -3.2, -7.2, 5.4, 6.7, -2.6, 6.4, 1.8 };
- CMatrix m1 = new CMatrix(da1);
- CMatrix m2 = new CMatrix(da2);
- CMatrix m3;
- CMatrix m4;
- m3 = m1 + m2;
- m4 = m1 * m2; // Matrix x Matrix
- m3.GebeWerteAus();
- Console.WriteLine("**************************************************");
- m4.GebeWerteAus();
- Console.WriteLine((double)m1); // Determinante von m1
- m1 = m1 * 4; // Matrix mit Skalar
- }
- }
- }